A. UDP stands for User Datagram Protocol. UDP provides an unreliable packet delivery system built on top of the IP protocol. As with IP, each packet is an individual, and is handled separately. Because of this, the amount of data that can be sent in a UDP packet is limited to the amount that can be contained in a single IP packet. Thus, a UDP packet can contain at most 65507 bytes (this is the 65535-byte IP packet size minus the minimum IP header of 20 bytes and minus the 8-byte UDP header).
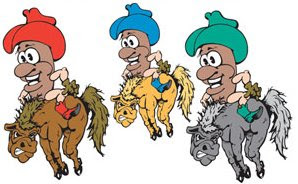
UDP packets can arrive out of order or not at all. No packet has any knowledge of the preceding or following packet. The recipient does not acknowledge packets, so the sender does not know that the transmission was successful. UDP has no provisions for flow control--packets can be received faster than they can be used. We call this type of communication connectionless because the packets have no relationship to each other and because there is no state maintained.
The destination IP address and port number are encapsulated in each UDP packet. These two numbers together uniquely identify the recipient and are used by the underlying operating system to deliver the packet to a specific process (application). Each UDP packet also contains the sender's IP address and port number.
One way to think of UDP is by analogy to communications via a letter. You write the letter (this is the data you are sending); put the letter inside an envelope (the UDP packet); address the envelope (using an IP address and a port number); put your return address on the envelope (your local IP address and port number); and then you send the letter.
Like a real letter, you have no way of knowing whether a UDP packet was received. If you send a second letter one day after the first, the second one may be received before the first. Or, the second one may never be received.
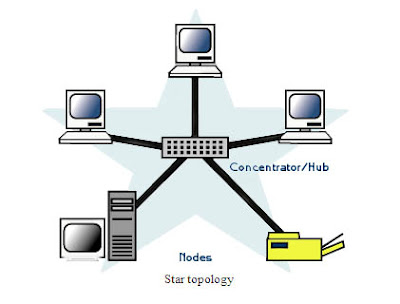
Q What is a Datagram?
A Datagram is another name for a UDP packet.
Java provides two classes for explicitly dealing with datagrams, DatagramSocket and DatagramPacket. These are both found in the java.net package.
Q Why use UDP if it is unreliable?
A. Two main reasons: speed and overhead.
UDP packets have almost no overhead--you simply send them then forget about them. And they are fast, because there is no acknowledgment required for each packet. Keep in mind that unreliable doesn't mean that packets can be lost or misdirected for no reason - it simply means that UDP provides no built-in checking and correction mechanism to gracefully deal with losses caused by network congestion or failure.
UDP is appropriate for the many network services that do not require guaranteed delivery. An example of this is a network time service. Consider a time daemon that issues a UDP packet every second so computers on the LAN can synchronize their clocks. If a packet is lost, it's no big deal--the next one will be by in another second and will contain all necessary information to accomplish the task.
Another common use of UDP is in networked, multi-user games, where a player's position is sent periodically. Again, if one position update is lost, the next one will contain all the required information.
A broad class of applications is built on top of UDP using streaming protocols. With streaming protocols, receiving data in real-time is far more important than guaranteeing delivery. Examples of real-time streaming protocols are real audio and real video which respectively deliver real-time streaming audio and video over the Internet. The reason a streaming protocol is desired in these cases is because if an audio or video packet is lost, it is much better for the client to see this as noise or "drop-out" in the sound or picture rather than having a long pause while the client software stops the playback, requests the missing data from the server. That would result in a very choppy, bursty playback which most people find unacceptable, and which would place a heavy demand on the server.
Q. What is Multicast and how does it work?
A. TCP and UDP are both unicast protocols; there is one sender and one receiver. Multicast packets are a special type of UDP packets. But while UDP packets have only one destination and only one receiver, multicast packets can have an arbitrary number of receivers.
Multicast is quite distinct from broadcast; with broadcast packets, every host on the network receives the packet. With multicast, only those hosts that have registered an interest in receiving the packet get it.
This is similar to the way an AWTEvent and its listeners behave in the Abstract Window Toolkit. In the same way that an AWTEvent is sent only to registered listeners, a multicast packet is sent only to members of the multicast group. AWT events, however, are unicast, and must be sent individually to each listener--if there are two listeners, two events are sent. With a MulticastSocket, only one is sent and it is received by many.
As you might guess, MulticastSocket is a subclass of DatagramSocket which has the extended ability to join and leave multicast groups. A multicast group consists of both a multicast address and a port number. The only difference between UDP and multicast in this respect is that multicast groups are represented by special internet addresses in the range 224.0.0.1 to 239.255.255.255, inclusive. Just as there are well-known ports for network services, there are reserved, well-known multicast groups for multicast network services.
When an application subscribes to a multicast group (host/port), it receives datagrams sent by other hosts to that group, as do all other members of the group. Multiple applications may subscribe to a multicast group and port concurrently, and they will all receive group datagrams.
When an application sends a message to a multicast group, all subscribing recipients to that host and port receive the message (within the time-to-live range of the packet, see below). The application needn't be a member of the multicast group to send messages to it.
Q What are RFCs and where can I find them?
A RFC stands for "Request for Comment". The RFCs form an integral part of the Internet standards; standards are formed by first publishing a specification as an RFC.
Q What is TCP and how does it work?
A Internet Protocol, or IP, provides an unreliable packet delivery system--each packet is an individual, and is handled separately. Packets can arrive out of order or not at all. The recipient does not acknowledge them, so the sender does not know that the transmission was successful. There are no provisions for flow control--packets can be received faster than they can be used. And packet size is limited.
Transmission Control Protocol (TCP) is a network protocol designed to address these problems. TCP uses IP, but adds a layer of control on top. TCP packets are lost occasionally, just like IP packets. The difference is that the TCP protocol takes care of requesting retransmits to ensure that all packets reach their destination, and tracks packet sequence numbers to be sure that they are delivered in the correct order. While IP packets are independent, with TCP we can use streams along with the standard Java file I/O mechanism.
Think of TCP as establishing a connection between the two endpoints. Negotiation is performed to establish a "socket", and the socket remains open throughout the duration of the communications. The recipient acknowledges each packet, and packet retransmissions are performed by the protocol if packets are missed or arrive out of order. In this way TCP can allow an application to send as much data as it desires and not be subject to the IP packet size limit. TCP is responsible for breaking the data into packets, buffering the data, resending lost or out of order packets, acknowledging receipt, and controlling rate of data flow by telling the sender to speed up or slow down so that the application never receives more than it can handle.
There are four distinct elements that make a TCP connection unique:
- IP address of the server
- IP address of the client
- Port number of the server
- Port number of the client
So a TCP connection is somewhat like a telephone connection; you need to know not only the phone number (IP address), but because the phone may be shared by many people at that location, you also need the name or extension of the user you want to talk to at the other end (port number). The analogy can be taken a little further. If you don't hear what the other person has said, a simple request ("What?") will prompt the other end to resend or repeat the phrase, and the connection remains open until someone hangs up.
Q. What's a MalformedURLException?
A. When you try to create a new URL by calling its constructor, it will throw a MalformedURLException if the URL string is not parseable or contains an unsupported protocol.
Q. How can I get the real local host IP address in an applet?
Applet security restrictions do not let you get this in an untrusted applet via InetAddress.getLocalHost().
However, you can get this address by creating a Socket connection back to the web server from which you came and asking the Socket for the local address:
URL url = getDocumentBase();
String host = url.getHost();
Socket socket = new Socket(host, 80);
InetAddress addr = socket.getLocalAddress();
String hostAddr = addr.getHostAddress();
System.out.println("Addr: " + hostAddr);
Q. What is the difference between a URI and a URL?
A. URLs are a subset of all URIs.
The term "Uniform Resource Locator" (URL) refers to the subset of URI that identify resources via a representation of their primary access mechanism (e.g., their network "location"), rather than identifying the resource by name or by some other attribute(s) of that resource.
No comments:
Post a Comment